Every language has a way to modify strings: add something to the end, search for something in the middle, concatenate two pieces of text, that sort of thing. C++ is no exception, and Unreal Engine exposes that functionality in Blueprint.
Let me give you an example: I wanted to find the name of a material I was using, then modify that name at the end, only to turn it back into a material via map lookup. This was for the Synty materials, many of which come with alternate options (xxx_A, xxx_B, etc). Hence I wanted to remove the A at the end and replace it with B. String operations made that possible.
Here’s how I did it:
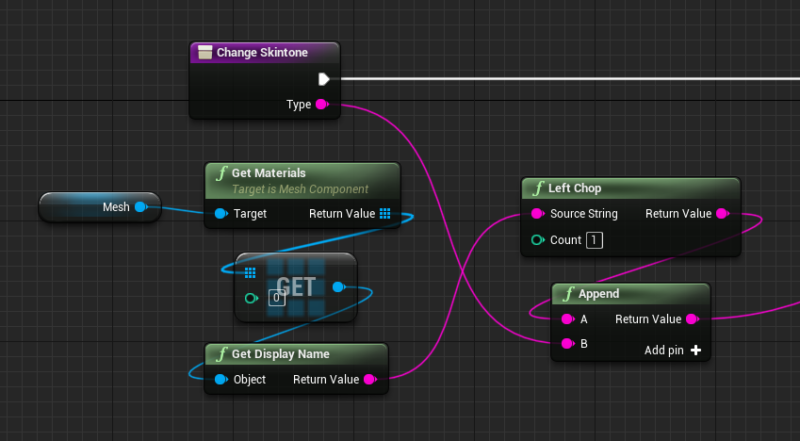
This is a function that takes one String value called Type. This will be a literal value like A, B or C. I’ll grab the first item in the array of materials from my mesh and Get its Display Name. That’s my input string value (something like ‘xxx_A’). The two important string related nodes here are Left Chop and Append: Left Chop takes my value and removes however many specified characters there are (Count value) to the left of it. In my case I need to lose 1, so I’m left with ‘xxx_’.
Append concatenates two or more strings. We’ll start with most of my initial value and add the A, B or C that was supplied on input, so we’ll get ‘xxx_B’ or ‘xxx_C’. Append can accept other pins that let us add several strings together.
There’s a whole host of other useful options at our disposal, taking me back to the Commodore days:
- Contains (test for substring inside a string)
- Ends With
- Mid (returns substring from position x for y characters)
- Parse Into Array (makes an array from long text separated by something)
- Reverse
- Right Chop
- Split
- Starts With
- Time Seconds to String
- To Lower
- To Upper
There are various other options too at our disposal too, here’s a full list of String related nodes from the official documentation. I’m sure they’ll come in handy when dealing with text.