The simplest way to animate a morph target directly in Sequencer is by writing a small helper function that sets the timeline’s value in the Tick Event. The morph targets themselves can be Shape Keys made in Blender, and their names will be retained. It is also possible to import morph targets into our mesh after the initial import. Let’s take a look at a simple example.
Creating my Object
For this demo I’m going to make a simple Icosphere, then setup a shape key and distort it a bit. This gives me a value of “Key 1” that when set, turns my sphere into a flat pancake.
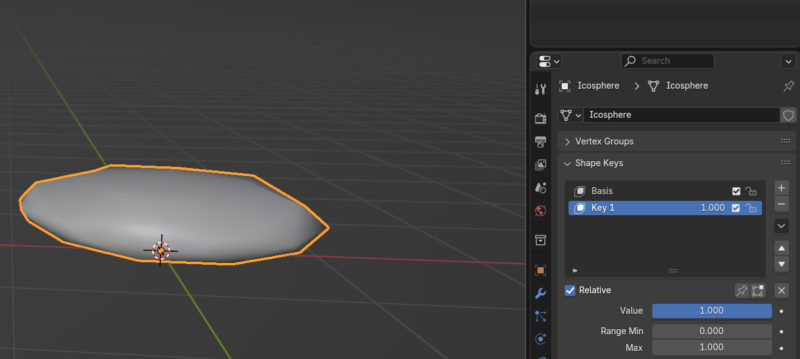
Let’s export this as FBX and bring it into Unreal Engine, then open it up in its Skeletal Mesh editor by double-clicking. Note that only Skeletal Meshes can be animated with morph targets properly, Static Meshes don’t support this feature (I mean they do, with a material hack, but it’s so terrible that’s easier not to even think about it).
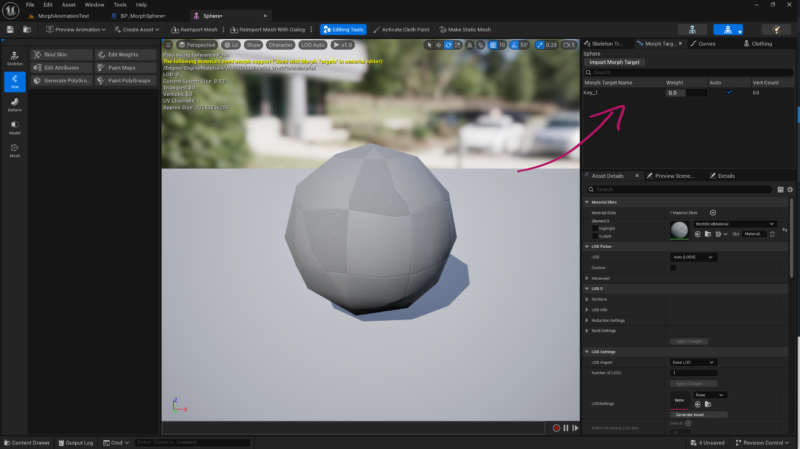
Notice our morph target at the top right. You can right-click and rename it here too. Also notice the “import morph target” button in the same section, with which you can bring in a morphed version of the same mesh and generate a new morph target. Make a mental note of the morph’s name (Key_1 in my case, not very descriptive), we’ll have to supply that in Blueprint code in a moment.
Building a Blueprint
To attach code to a skeletal mesh, we’ll need to create a Blueprint. A simple actor class will do, then we’ll add a Skeletal Mesh Component to it and add our sphere mesh. We’ll also need two float variables: one public (the value we’re animating, I’ll call mine Flat Morph), and another internal one I’ll call Flat Current.
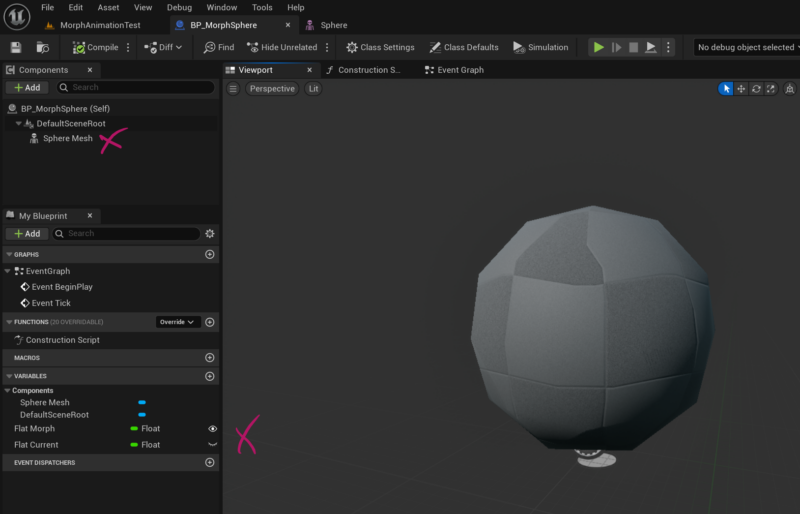
On the public variable, enable “Expose to Cinematics” so that we can animate the value via Sequencer.
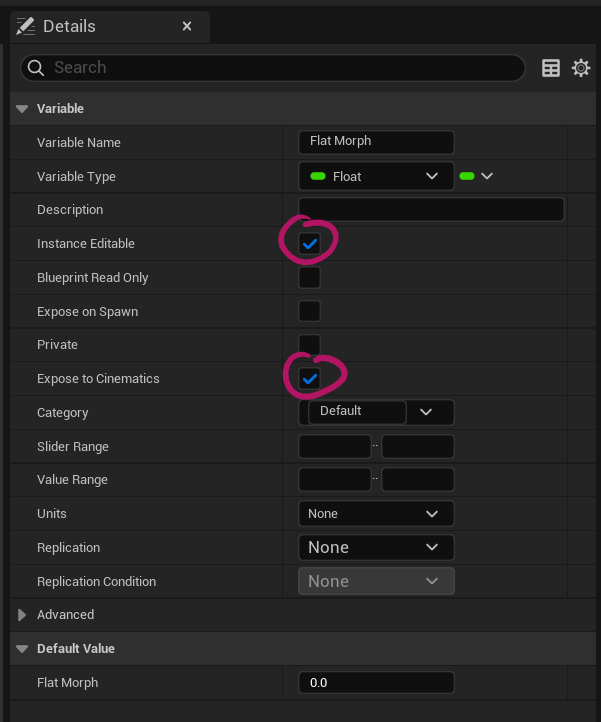
Although we can now track our Blueprint on a Level Sequence and set values on our variable, nothing is happening yet. That’s because of two things, the first of which is that some code is missing we’ll have to write. We want to find out what the current value of our morph is, compare it to whatever the Sequencer tells us it should be, and if a change is necessary, make that change. The Tick event is just the place for this logic:
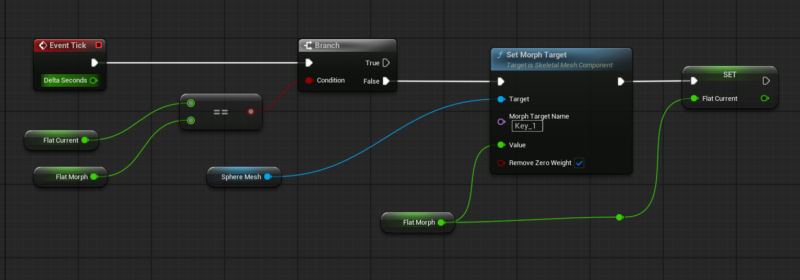
Here we compare the external (cinematic) variable to our internal current value, and if they deviate (false branch), we set the new value on the morph target. We’ll also balance our variables at the end so that they’re the same.
Speaking of which, we need to give the second variable a starting value for good measure, although it’s not essential in the context of cinematics (might be a bit nicer on playback though). We’ll do that in Begin Play:
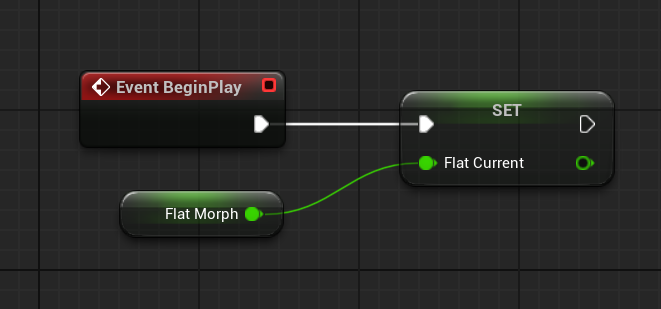
Animate the value in Sequencer
Let’s add a Level Sequence and track our Sphere Blueprint on it. Click the plus icon next to the Blueprint and select your variable name, then go mad and set keyframes. As you move the play head, you’ll see the values change. That’s a good start!
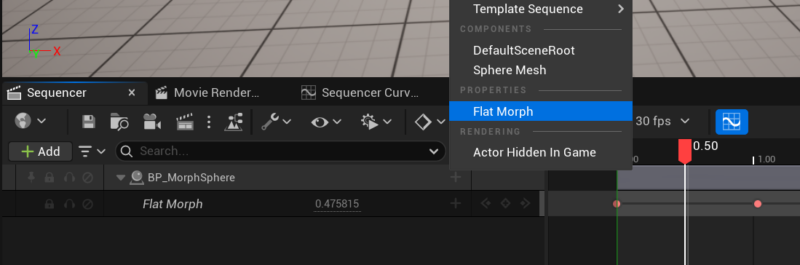
Nothing is happening to the object yet, but it will when you start rendering the sequence. This is because the Tick Even is called during rendering on every frame (much like if it were real time game play), but it isn’t called in regular editor mode. To alleviate this and preview our animation so that we can make meaningful changes, switch into Simulate Mode. Unreal Engine 5.5 has introduced a super handy one-click option for that (in 5.4 and lower you need to click on the three little dots and change this over from the drop-down menu).
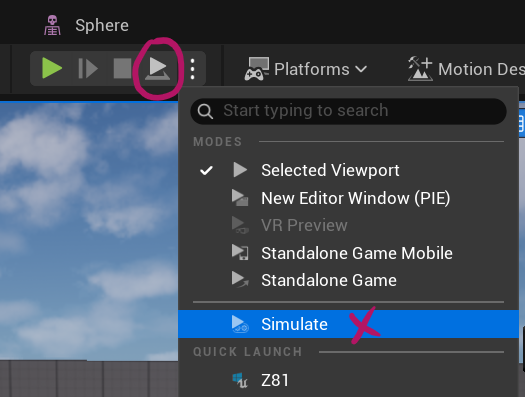
Now move the play head and see how the shape of your object changes as if by magic.